mirror of
https://github.com/ocornut/imgui.git
synced 2024-10-16 08:15:14 -05:00
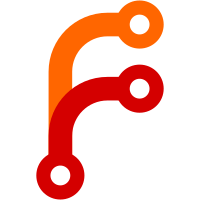
Currently, the implementation headers don't include the imgui.h header. Which means that the compilation will fail if the implementation header was included before the imgui.h header in the compilation unit. For instance, a compilation unit with the following will work: #include "imgui.h" #include "imgui_impl_glfw.h" #include "imgui_impl_opengl3.h" But a compilation unit with the following will fail because IMGUI_IMPL_API and possibly other symbols will not be defined: #include "imgui_impl_glfw.h" #include "imgui_impl_opengl3.h" #include "imgui.h" This patch includes imgui.h in the implementation headers to make inclusions order-invariant, which is a recommended practice.
32 lines
1.8 KiB
C
32 lines
1.8 KiB
C
// dear imgui: Renderer for OpenGL2 (legacy OpenGL, fixed pipeline)
|
|
// This needs to be used along with a Platform Binding (e.g. GLFW, SDL, Win32, custom..)
|
|
|
|
// Implemented features:
|
|
// [X] Renderer: User texture binding. Use 'GLuint' OpenGL texture identifier as void*/ImTextureID. Read the FAQ about ImTextureID!
|
|
|
|
// You can copy and use unmodified imgui_impl_* files in your project. See main.cpp for an example of using this.
|
|
// If you are new to dear imgui, read examples/README.txt and read the documentation at the top of imgui.cpp.
|
|
// https://github.com/ocornut/imgui
|
|
|
|
// **DO NOT USE THIS CODE IF YOUR CODE/ENGINE IS USING MODERN OPENGL (SHADERS, VBO, VAO, etc.)**
|
|
// **Prefer using the code in imgui_impl_opengl3.cpp**
|
|
// This code is mostly provided as a reference to learn how ImGui integration works, because it is shorter to read.
|
|
// If your code is using GL3+ context or any semi modern OpenGL calls, using this is likely to make everything more
|
|
// complicated, will require your code to reset every single OpenGL attributes to their initial state, and might
|
|
// confuse your GPU driver.
|
|
// The GL2 code is unable to reset attributes or even call e.g. "glUseProgram(0)" because they don't exist in that API.
|
|
|
|
#pragma once
|
|
|
|
#include "imgui.h"
|
|
|
|
IMGUI_IMPL_API bool ImGui_ImplOpenGL2_Init();
|
|
IMGUI_IMPL_API void ImGui_ImplOpenGL2_Shutdown();
|
|
IMGUI_IMPL_API void ImGui_ImplOpenGL2_NewFrame();
|
|
IMGUI_IMPL_API void ImGui_ImplOpenGL2_RenderDrawData(ImDrawData* draw_data);
|
|
|
|
// Called by Init/NewFrame/Shutdown
|
|
IMGUI_IMPL_API bool ImGui_ImplOpenGL2_CreateFontsTexture();
|
|
IMGUI_IMPL_API void ImGui_ImplOpenGL2_DestroyFontsTexture();
|
|
IMGUI_IMPL_API bool ImGui_ImplOpenGL2_CreateDeviceObjects();
|
|
IMGUI_IMPL_API void ImGui_ImplOpenGL2_DestroyDeviceObjects();
|